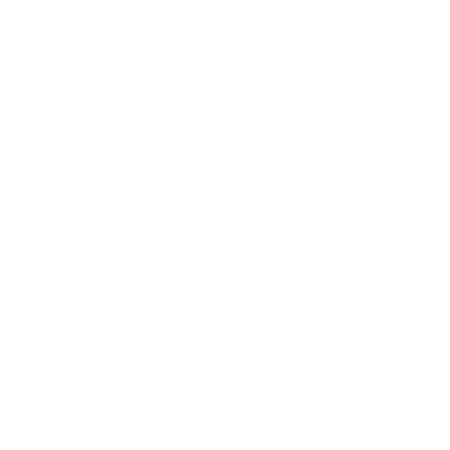
Darum sind Sie bei uns richtig
Ihr Partner für erstklassige Softwarelösungen und hervorragende Beratung!
Unsere innovativen und massgeschneiderten Lösungen sind darauf ausgerichtet, Ihnen dabei zu helfen, Ihre Ziele zu erreichen. Mit langjähriger Erfahrung und fundiertem Fachwissen bieten wir hochwertige Dienstleistungen an.
Unsere nachgewiesene Erfolgsbilanz und unsere Hingabe zur Exzellenz haben uns als vertrauenswürdigen Partner für viele Konsumenten etabliert. Bei der WebGate stehen wir Ihnen zur Seite, um Ihre technologischen Ziele zu realisieren und Ihr Geschäft auf die nächste Stufe zu heben. Hier sind Sie genau richtig, um die bestmögliche Unterstützung für Ihre Anforderungen zu erhalten.
Unsere Werte
LOYAL
ZUVERLÄSSIG
FLEXIBEL
ANTIZIPIEREND
INNOVATIV
Frische Ansätze
Unsere Projekte zeichnen sich durch die kontinuierliche Suche nach frischen, innovativen Ideen aus. Das Ergebnis sind verbesserte Lösungen mit einem erheblichen Mehrwert für unsere Kunden.
Business Know-how
Ob Digitalisierung, Industrie 4.0 oder Content Management – für uns liegt der Schlüssel zum Erfolg in der digitalen Kernkompetenz eines Unternehmens. Wir unterstützen unsere Kunden tatkräftig bei der Umsetzung.
Benutzerfreundlich gestaltet
In einer zunehmend digitalen Geschäftswelt verlagern sich immer mehr Prozesse in den digitalen Kern von Unternehmen. Daher ist eine intuitive Benutzeroberfläche wichtiger denn je. Wir sehen eine detaillierte Gestaltung der Benutzererfahrung als entscheidenden Schritt für reibungslose Abläufe.
Innovation durch Design
Die IT wandelt sich rasch. Wir halten stets Ausschau nach den aktuellsten Markttrends und prüfen sorgfältig, ob eine Integration für unsere Kunden vorteilhaft ist.
Prozessoptimierung
Wiederkehrende Abläufe werden von uns in hochdynamische Software eingebettet. Dies gewährleistet nicht nur einen einheitlichen Qualitätsstandard, sondern spart Ihren Mitarbeitenden auch langfristig viel Aufwand.
Systemintegration
Unsere Lösungen fügen sich mühelos in ein Gesamtkonzept ein und sind nicht isoliert. Wir etablieren Verbindungen zwischen Systemen, um einen reibungslosen Datenfluss zu gewährleisten.